Superlight vanilla JS dropdowns
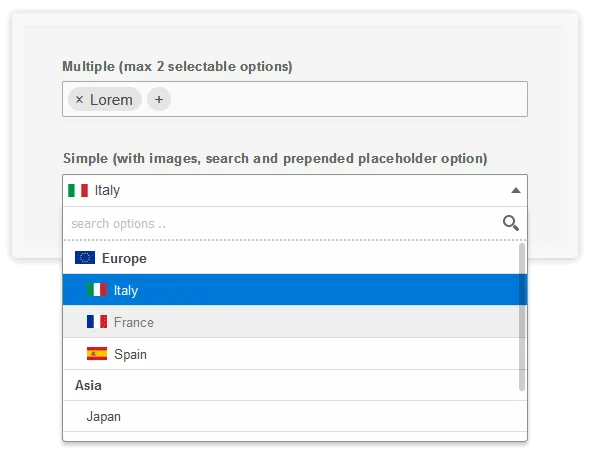
- sigle 18KB file, no dependencies, 100% pure javascript
- two themes (light, dark) included. Designed to be themed with no efforts
- themes mix support using prefixed selectors
- supports both simple and multiple select fields
- fully responsive, fits in any width
- (optional) default placeholder for simple select fields
- (optional) searchbar with minimum fields threshold
- (optional) maximum selectable options
- complete keyboard events integration
- option images support
- mobile ready
- multilanguage ready
Basic Implementation
Full-feature Implementation
Mixing themes
The easiest and cleanest way to mix themes on the same webpage is
- using addit_classes option to specify the theme prefix
- include prefixed themes version (there are prefixed versions of dark/ligh themes in the “themes” folder)
Extras
There are two extra events you can trigger on initialized elements:
lc-select-refresh: re-sync select field options and status with plugin instance (eg. when new fields are dynamically added)
lc-select-destroy: remove plugn instance, coming back to HTML select field